As a follow up to the Naughts and Crosses game, our next programming challenge in school was a console based Connect 4 game. This game took similar logic from the Naughts and Crosses game and expanded on it. We now have a 7x6 grid to place counters in, counters must fall into the correct position (cannot levitate) and four in a row must be achieved this time (instead of three in N&C). This posed some brand new challenges.
The design of the board is made using another 2D array and we use a nested loop to print the board based on the values stored in that array. The board looks as followed:
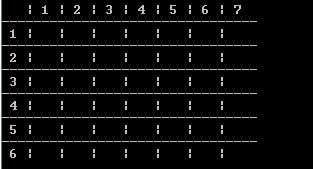
We do not actually need the row labels since a user only has to decide what column they would like to place their counter in however this helps make the board look more appealing.
Again we have a while loop which controls the main flow of the program. This while loop continuously switches the player turn whilst checking for a win or draw on every turn. On each players’ go, they are able to select which column to drop their counter in and providing it is not already full, the counter will drop to the lowest available space in that column. A for loop is used to calculate this lowest space.
The check for win algorithm is deceptively challenging when it comes to the diagonals. The horizontal and vertical check use some simple for loops to check multiple vertical/horizontal runs quickly. My algorithm to check for diagonals would firstly split the board into diagonal lines (e.g. (1,4), (2,3), (3,2) and (4,1)). If the diagonal lines were only a length of four, it would check the one possible run of four. However if the lines were five spaces long, it would loop twice, doing the two possible runs of four. It would loop three times for the runs of six. It would also do this for both directions (bottom left to top right diagonals and bottom right to top left diagonals). This is quite difficult to explain but needless to say the solution algorithm we looked at in class was much more efficient. I stuck with my algorithm though, since it was my solution to the problem and it worked.
Once this was finished, the game worked well and the end result looked like this:
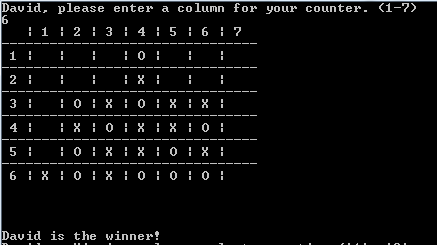
I then implemented the same save and loading options which would read and write to a textfile to store the selected games. This was challenging with the larger data amount however I managed to make it work in the end. Again it is the winner name (or player one name if it is a draw) and the date which act as a key to find the game.
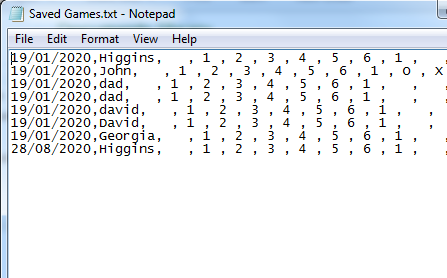